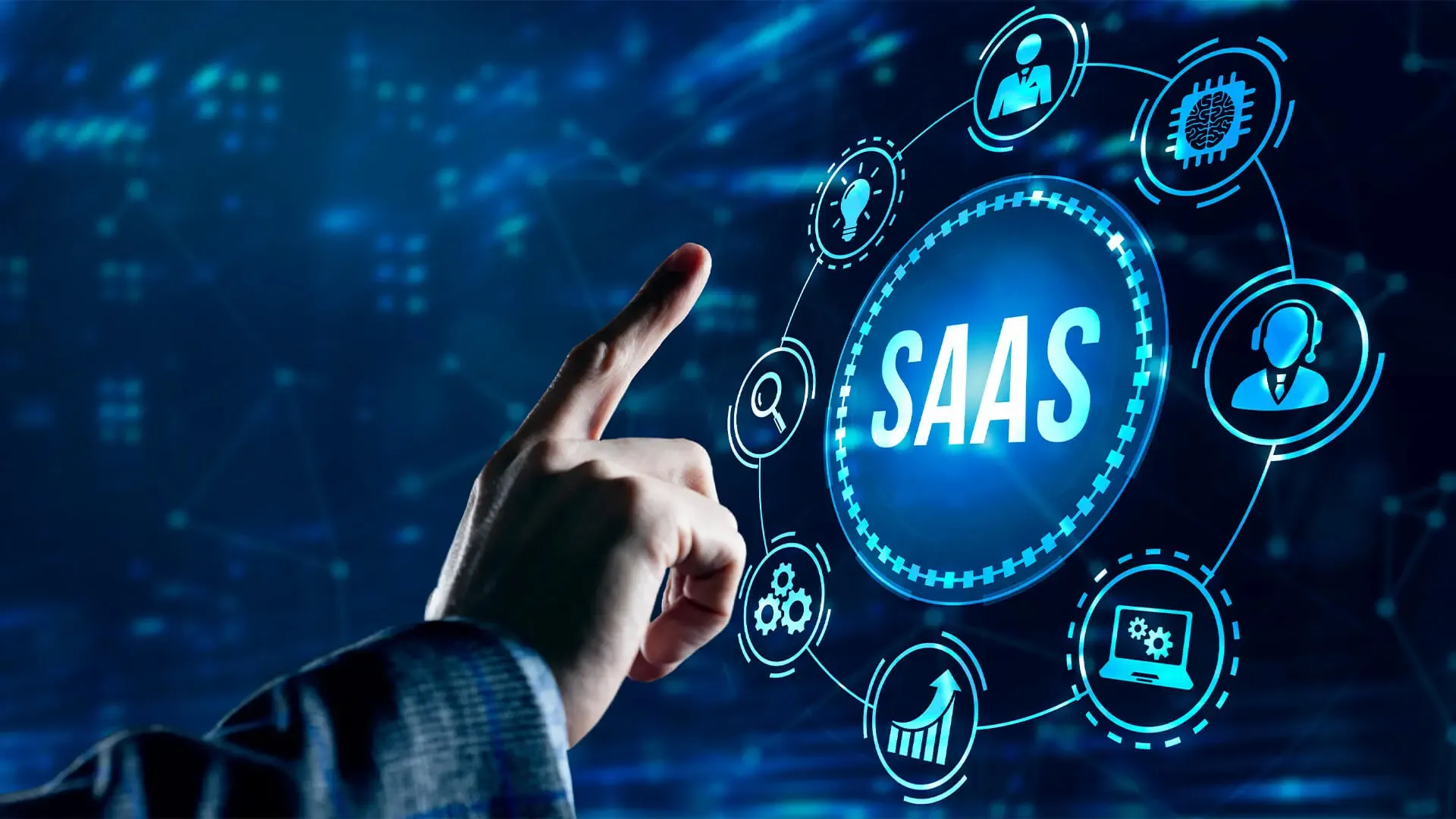
Scalable architecture from the ground up
Developing software as a service (SaaS) comes with the challenge of designing an architecture that can scale as your user base grows. A scalable architecture ensures your application remains reliable, high-performing, and maintainable even with increasing load. In this article, we’ll explore how to build a scalable architecture from the beginning, focusing on real-world patterns and best practices. We’ll look at the trade-offs between monolithic vs. microservices designs, the role of event-driven architectures, strategies for database scalability, and techniques for performance optimization. Along the way, we’ll draw examples from SaaS companies that successfully scaled their systems and reference expert advice from books like Microservices Patterns by Chris Richardson and insights from Kent Beck’s works.
Monolith vs. Microservices: Laying the Foundation
One of the first big decisions is whether to start with a monolithic architecture or break the system into microservices. A monolith is a single unified codebase and deployable unit, while a microservices architecture consists of many small, independent services communicating over APIs. Each approach has pros and cons:
Monolithic Architecture
- Simpler to start and deploy as one package.
- All components (UI, backend logic, database access) reside in one codebase and run together.
- Can accelerate early development and avoid the operational overhead of managing many services.
- However, as the application grows, a monolith can become a “big ball of mud” – tightly coupled components that are hard to maintain or scale independently.
Even large companies like Amazon and Netflix started with monoliths before transitioning to microservices.
Microservices Architecture
- Breaks the application into multiple services, each responsible for a specific business capability.
- Services can be developed, deployed, and scaled independently.
- Improves agility and fault isolation: a change or failure in one service ideally doesn’t bring down the whole system.
- Many web-scale companies (Netflix, Amazon, eBay, Uber, etc.) evolved from monoliths to microservices.
- However, managing a distributed system comes with complexity (e.g., service communication, data consistency, observability).
Best Approach? Start With a Modular Monolith
According to Martin Fowler, “almost all the successful microservice stories have started with a monolith that got too big and was broken up.” Kent Beck’s philosophy “Make it work, make it right, make it fast” aligns with this—build a functional solution first, refine its design, and optimize for scale when necessary.
A modular monolith allows for:
- Clear separation between components within the monolith.
- Easier migration to microservices if needed (following the Strangler Fig pattern).
- Avoiding premature complexity from microservices overhead.
Event-Driven Architecture for Decoupling
No matter which high-level architecture you choose, adopting an event-driven architecture (EDA) can improve scalability and flexibility in a SaaS application.
How Event-Driven Systems Work
Instead of direct API calls between services, components communicate asynchronously by emitting and reacting to events. Examples:
- Traditional request-response: Service A calls Service B’s API and waits for a response.
- Event-driven: Service A emits an event, and Service B (or multiple services) react to it asynchronously.
Benefits of Event-Driven Architecture
- Decoupling: Services don’t need to know about each other, making it easier to scale and modify the system.
- Resilience: If one service fails, others can continue processing events later.
- Elasticity: Event queues buffer spikes in workload (e.g., handling thousands of orders in a flash sale).
Real-World Example: Airbnb & Netflix
- Airbnb uses Kafka-based event pipelines for real-time analytics and system monitoring.
- Netflix uses event-driven architecture to process billions of data points across microservices efficiently.
Database Scalability and Multi-Tenancy
For SaaS applications, the database is often the hardest part of the stack to scale. Common strategies include:
Vertical Scaling vs. Horizontal Scaling
- Vertical scaling: Upgrading the database server (CPU, RAM, SSDs). Simple but expensive.
- Horizontal scaling: Distributing data across multiple nodes or databases.
Scaling Strategies
- Read Replicas: Reduce load on the main database by creating read-only replicas.
- Partitioning (Sharding): Split data into smaller databases based on user ID, tenant, or other keys.
- Caching (Redis, Memcached): Store frequently accessed data in memory to improve performance.
- Polyglot Persistence (NoSQL + SQL): Use different databases for different workloads (e.g., relational DB for transactions, NoSQL for logs/analytics).
Multi-Tenant SaaS Considerations
- Shared Database, Shared Schema: All tenants share the same tables (simpler but requires strict data isolation).
- Separate Databases per Tenant: Strong isolation but harder to manage at scale.
- Hybrid Model: Use shared schema for smaller tenants, dedicated databases for larger customers (e.g., Slack uses MySQL sharding with Vitess).
Performance Optimization and Best Practices
1. Stateless Services & Auto-Scaling
- Keep application servers stateless so they can be easily scaled horizontally.
- Store session data in Redis or a distributed store instead of local memory.
2. Caching at Multiple Levels
- Database Caching: Reduce repeated queries with Redis or Memcached.
- Application Caching: Store API responses for frequently accessed data.
- Edge Caching (CDN): Deliver static content faster with Cloudflare, AWS CloudFront, or Fastly.
3. Asynchronous Processing & Background Jobs
- Move non-essential work (e.g., sending emails, data processing) to background jobs.
- Use job queues (RabbitMQ, Kafka, Celery, Sidekiq) to handle delayed execution.
4. Load Balancing & Auto-Scaling
- Distribute incoming requests across multiple instances with a load balancer.
- Set up auto-scaling groups to dynamically adjust capacity based on demand.
5. Profiling & Performance Tuning
- Use APM tools (Datadog, New Relic) to identify bottlenecks.
- Optimize slow database queries, add indexes where necessary.
- Follow Kent Beck’s principle: “Make it right, then make it fast.”
Further Reading
To dive deeper into scalable software architecture, check out these resources:
- Microservices Patterns – Chris Richardson
- Monolith to Microservices – Sam Newman
- Martin Fowler’s Blog: MonolithFirst
- Kent Beck’s Extreme Programming Explained
- Netflix Tech Blog: Microservices & Scaling Challenges
- Slack Engineering: Scaling Datastores with Vitess
- Designing Data-Intensive Applications – Martin Kleppmann
Articles
Serverless computing: is it the future of scalable applications?
What is serverless computing and how does it help build scalable applications? Pros, cons, applications and an overview of the major platforms.
Engineering approach in practice - CraftByte Studio standards
How we develop software with engineering precision: architecture, CI/CD, integration testing. No buzzwords, no rubbish. Check.
Scalable architecture from the ground up
Learn how to build a scalable SaaS architecture with best practices in microservices, event-driven design, database scaling, and performance optimization.
How to Effectively Automate Business Processes with Software
Discover how business process automation can streamline workflows, reduce costs, and improve efficiency using AI, RPA, and workflow automation tools.
Key Global IT Trends Shaping the Future of Business
Top IT Trends 2025! AI, Automation, Cloud, Cybersecurity & the Future of Technology Shaping Innovation in Modern Business!
Specialist vs. Generalist in IT
Specialists excel in deep expertise, while generalists thrive on adaptability. The best developers blend both for long-term success in an AI-driven world.
Custom Software: When Does It Make Sense?
Struggling with off-the-shelf software limitations? Discover when custom solutions make sense for scalability, efficiency, and long-term savings.